How To Display Json Data In Html
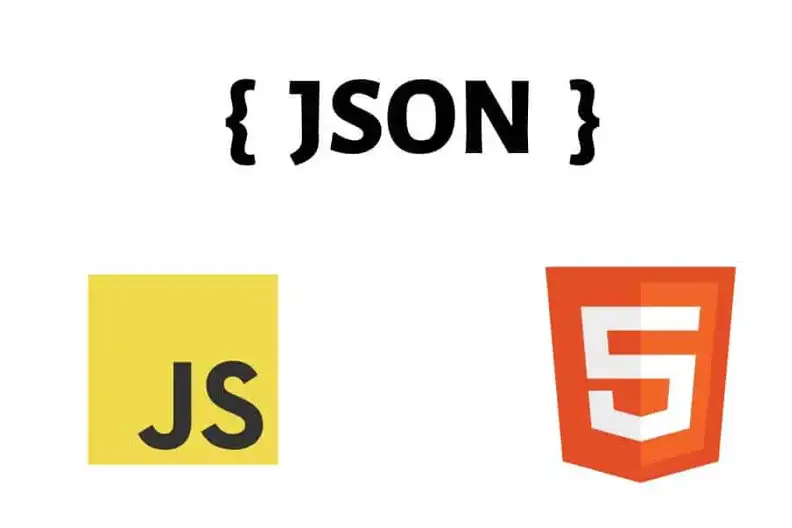
In this tutorial, I will show you how to fetch and display information from a JSON file using vanilla JavaScript.
Then how will we achieve this?
Starting time, nosotros volition fetch the JSON data by using the fetch API. This will render a promise with our JSON information. Then we will append the data dynamically by creating HTML elements on the fly. We will then append our JSON data to those elements.
Getting JSON data from an API and display information technology on a spider web folio is a common thing you will do quite often. I have created similar posts on the large frameworks similar React, Vue and Angular. Check it out if you are using any of those frameworks.
Let'south get started!
First, create a people.json
file and fill up it with the following data:
[ { "id": "ane", "firstName": "John", "lastName": "Doe" }, { "id": "two", "firstName": "Mary", "lastName": "Peterson" }, { "id": "iii", "firstName": "George", "lastName": "Hansen" } ]
We volition save this file in the same directory as our alphabetize.html
file.
Fetching the JSON information
To be able to display this information in our HTML file, we starting time demand to fetch the data with JavaScript.
We volition fetch this data by using the fetch API. We utilise the fetch API
in the following style:
fetch(url) .and so(office (response) { // The JSON information will arrive here }) .take hold of(function (err) { // If an error occured, y'all will take hold of it here });
url
fetch
people.json
index.html
The fetch
function will return a hope. When the JSON data is fetched from the file, the then
role will run with the JSON information in the response.
If annihilation goes wrong (like the JSON file cannot be found), the catch
part will run.
Let us see how this will look in out case:
fetch('people.json') .then(function (response) { render response.json(); }) .and so(function (information) { appendData(information); }) .catch(office (err) { panel.log(err); });
Here we are fetching our people.json
file. After the file has been read from disk, we run the then
part with the response as a parameter. To get the JSON data from the response, we execute the json()
function.
The json()
office likewise returns a promise. This is why we just render it and chain another then
role. In the second and so
function nosotros get the actual JSON data as a parameter. This data looks only like the information in our JSON file.
Now we tin can take this data and display information technology on our HTML page. Find that we are calling a role called appendData
. This is where we create the lawmaking which will append the data to our folio.
Notice that in our catch
function, we are just writing the error message to out console. Commonly you would display an error message to the user if something went wrong.
Displaying the JSON data
Before nosotros brandish our JSON data on the webpage, let's just see how the torso of our alphabetize.html
file looks like.
<body> <div id="myData"></div> </trunk>
Pretty simple right? We have just a elementary div with the id myData
. Our plan is to fill our JSON data inside this div dynamically.
There are several ways to display the data in our HTML. We could create a table and brand it look actually good with nice styling. Nevertheless, we will do this in a elementary and ugly style.
Our goal is to just simply display the full proper noun of the people in our JSON file.
Step 1 – Get the div element from the body
Remember the div with the myData
id from index.html
This is how we will practise it:
var mainContainer = document.getElementById("myData");
Nosotros get the element by executing the getElementByID
office. This will find the chemical element with the id myData
. This is vanilla JavaScript and this is how nosotros did front-end evolution in the "quondam" days :).
Pace 2 – Loop through every object in our JSON object
Next step is to create a simple loop. We can then go every object in our listing of JSON object and append it into our chief div.
for (var i = 0; i < information.length; i++) { // append each person to our folio }
Step 3 – Suspend each person to our HTML page
Inside the for-loop we will append each person inside its own div. This code volition be repeated three times for each person.
Get-go, we will create a new div chemical element:
var div = document.createElement("div");
Next we volition make full that div with the total proper name from our JSON file.
div.innerHTML = 'Name: ' + data[i].firstName + ' ' + data[i].lastName;
Lastly, we will append this div to our main container:
mainContainer.appendChild(div);
That'south it. Now nosotros take finished appending the JSON data to our index.html folio. The full appendData
function looks like this:
function appendData(data) { var mainContainer = document.getElementById("myData"); for (var i = 0; i < information.length; i++) { var div = document.createElement("div"); div.innerHTML = 'Proper noun: ' + data[i].firstName + ' ' + data[i].lastName; mainContainer.appendChild(div); } }
When we run our index.html folio, it will look something like this:
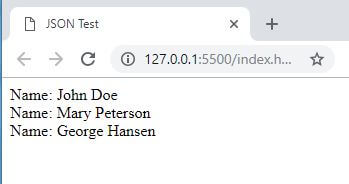
Not the nearly beautiful application, only it got the job done.
Permit us look at the entire HTML file with the JavaScript:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-eight"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Uniform" content="ie=border"> <title>JSON Test</championship> </head> <trunk> <div id="myData"></div> <script> fetch('people.json') .then(role (response) { return response.json(); }) .then(office (information) { appendData(data); }) .grab(office (err) { console.log('error: ' + err); }); function appendData(data) { var mainContainer = certificate.getElementById("myData"); for (var i = 0; i < information.length; i++) { var div = document.createElement("div"); div.innerHTML = 'Name: ' + data[i].firstName + ' ' + data[i].lastName; mainContainer.appendChild(div); } } </script> </body> </html>
Try to copy and paste this in your own editor. As an do, you can try to style the output to look nicer. Remember to include the people.json
file as well. This file must be in the same directory as your index.html
file for this to work.
Why use Vanilla JavaScript?
You might be wondering what is the point of creating this in vanilla JavaScript. Doesn't modern web application use frameworks and libraries like Angular, ReactJS or VueJS?
Well, yeah, you lot are probably correct, about of the fourth dimension. But some web pages are just static with very trivial logic.
If you lot just want to tweak some modest parts of the website, it might exist overkill to include big libraries which will slow down the site.
Besides, frameworks and libraries come and go. Good old vanilla JavaScript is here to stay. So take every opportunity to learn it, you don't know when you might demand it.
Happy coding!
How To Display Json Data In Html,
Source: https://howtocreateapps.com/fetch-and-display-json-html-javascript/
Posted by: bennettweland.blogspot.com
0 Response to "How To Display Json Data In Html"
Post a Comment